Button
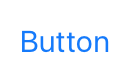
super: UIButton (on iOS)
A Button object is a view that executes your custom code in response to user interactions. You communicate the purpose of a button using a text label, an image, or both.
Events
-
Load() This event is called when the object becames available in the current runtime system.
-
WillShow() The view is about to be added to the App's views hierarchy.
-
WillHide() The view is about to be removed from the App's views hierarchy.
-
Action() This event is called when a touch is released (touch-up) inside the bounds of the button
-
DidShow() The view has been added to the App's views hierarchy.
-
DidHide() The view has been removed from the App's views hierarchy.
-
Unload() This event is called when the object has been removed from the current runtime system (but not yet deallocated).
Properties
-
var title: String The title of the button. Use this property instead of the titleForState and setTitleForState methods if you want to use the same title for all the button states.
-
var font: Font The font used for the button’s title string.
-
var type: ButtonType Returns the button type. (read-only)
-
var currentTitle: String Returns the title for the current button state. (read-only)
-
var currentTitleColor: Color Returns the color of the title for the current button state. (read-only)
-
var currentTitleShadowColor: Color Returns the color of the title shadow for the current button state. (read-only)
-
var currentImage: Image Returns the image for the current button state. (read-only)
-
var currentBackgroundImage: Image Returns the background image for the current button state. (read-only)
-
var objectName: String The name of the object.
Constructors
- func Button(buttonType: ButtonType) Creates and returns a new button of the specified type.
Methods
-
func titleForState(state: ControlState): String Returns the title associated with the specified state.
-
func setTitleForState(title: String, state: ControlState) Sets the title associated with the specified state. At a minimum, you should set the value for the normal state. If a title is not specified for a state, the default behavior is to use the title associated with the normal state. If the value for normal is not set, then the property defaults to a system value.
-
func titleColorForState(state: ControlState): Color Returns the title color used for the specified state.
-
func setTitleColorForState(color: Color, state: ControlState) Sets the color of the title to use for the specified state.
-
func titleShadowColorForState(state: ControlState): Color Returns the shadow color of the title used for the specified state.
-
func setTitleShadowColorForState(color: Color, state: ControlState) Sets the color of the title shadow to use for the specified state.
-
func imageForState(state: ControlState): Image Returns the image used for the specified state.
-
func setImageForState(image: Image, state: ControlState) Sets the image to use for the specified state.
-
func backgroundImageForState(state: ControlState): Image Returns the background image used for the specified state.
-
func setBackgroundImageForState(image: Image, state: ControlState) Sets the background image to use for the specified state.
-
func animate(duration: Float, delay: Float, options: AnimationOption, animations: Closure, completion: Closure) Animate changes to one or more views using the specified duration, delay, options and completion handler.
-
func setFocus() Force focus to be set to the selected control. For TextField and TextView that means force Keyboard to appear.
-
func clearFocus() Clear focus from selected control
Enums
ButtonType
- .ContactAdd
- .Custom
- .DetailDisclosure
- .InfoDark
- .InfoLight
- .RoundedRect
- .System
ControlState
- .Application
- .Disabled
- .Focused
- .Highlighted
- .Normal
- .Selected
AnimationOption
- .AllowAnimatedContent
- .AllowUserInteraction
- .Autoreverse
- .BeginFromCurrentState
- .CurveEaseIn
- .CurveEaseInOut
- .CurveEaseOut
- .CurveLinear
- .LayoutSubviews
- .OverrideInheritedCurve
- .OverrideInheritedDuration
- .OverrideInheritedOptions
- .Repeat
- .ShowHideTransitionViews
- .TransitionCrossDissolve
- .TransitionCurlDown
- .TransitionCurlUp
- .TransitionFlipFromBottom
- .TransitionFlipFromLeft
- .TransitionFlipFromRight
- .TransitionFlipFromTop
- .TransitionNone
Examples
// How to programmatically stretch a background image to fill a button background
// Add this code in the button Load() function.
// Load a generic button background image, it's middle content can be stretched but not the corners!
var img = Image("button_image");
// Create an EdgeInset according to the button's shape to "cut-out" the corners (20x20 is a size measured in points)
var edge = EdgeInsets(20, 20, 20, 20);
// Create a new image with the loaded button background and the properly set EdgeInsets
img = img.resizableImageWithCapInsets(edge, ImageResizingMode.Stretch);
// Set the image as the new background image for the current button
self.setBackgroundImageForState(img, ControlState.Normal);