MapView
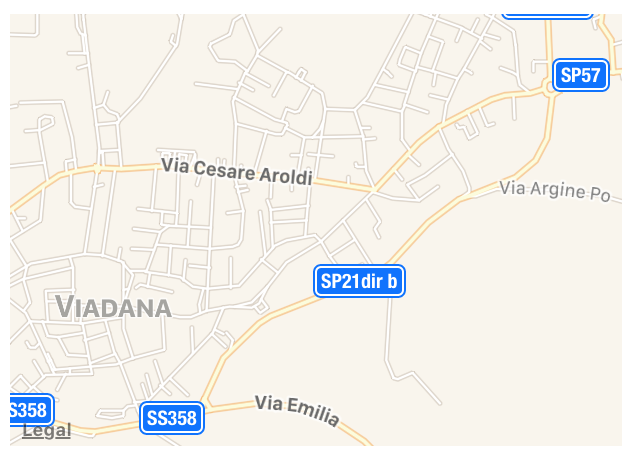
super: UIView (on iOS)
Display map or satellite imagery from the windows and views of your custom apps.
Events
-
Load() This event is called when the object becames available in the current runtime system.
-
WillShow() The view is about to be added to the App's views hierarchy.
-
WillHide() The view is about to be removed from the App's views hierarchy.
-
WillStart() Tells that the map view will start tracking the user's position.
-
DidFail() Tells that an attempt to locate the user’s position failed.
-
DidStop() Tells that map view stopped tracking the user’s location.
-
LocationDidChange(value: Map) The location of the user was updated.
-
DidSelectAnnotation(annotationPoint: MapPointAnnotation) Raised when one of its annotation views was selected.
-
DidDeselectAnnotation(annotationPoint: MapPointAnnotation) Raised when one of its annotation views was deselected.
-
DidShow() The view has been added to the App's views hierarchy.
-
DidHide() The view has been removed from the App's views hierarchy.
-
Unload() This event is called when the object has been removed from the current runtime system (but not yet deallocated).
Properties
-
var address: String A string describing the location you want to center the map to.
-
var latitude: Float The latitude of the center point of the visible area.
-
var longitude: Float The longitude of the center point of the map.
-
var mapType: MapType The type of data displayed by the map view.
-
var zoomValue: Float The zoom value used to display the map.
-
var zoomEnabled: Bool A Boolean value that determines whether the user may use pinch gestures to zoom in and out of the map.
-
var scrollEnabled: Bool A Boolean value that determines whether the user may scroll around the map.
-
var pitchEnabled: Bool A Boolean value indicating whether the map camera’s pitch information is used.
-
var rotateEnabled: Bool A Boolean value indicating whether the map camera’s heading information is used.
-
var visibleMapRect: Rect The area currently displayed by the map view.
-
var showsPointsOfInterest: Bool A Boolean indicating whether the map displays point-of-interest information.
-
var showsBuildings: Bool A Boolean indicating whether the map displays extruded building information.
-
var showsUserLocation: Bool A Boolean value indicating whether the map should try to display the user’s location.
-
var userLocationVisible: Bool A Boolean value indicating whether the device’s current location is visible in the map view. (read-only)
-
var userTrackingMode: MapUserTrackingMode Sets the mode used to track the user location.
-
var annotationVisibleRect: Rect The visible rectangle where annotation views are currently being displayed. (read-only)
-
var annotations: List The complete list of annotations associated with the map. (read-only)
-
var selectedAnnotations: List The annotations that are currently selected. Assigning a new array to this property selects only the first annotation in the array.
-
var objectName: String The name of the object.
Methods
-
func addAnnotation(annotationPoint: MapPointAnnotation) Adds the specified annotation to the map view.
-
func removeAnnotation(annotationPoint: MapPointAnnotation) Removes the specified annotation object from the map view.
-
func annotationsInRect(regionRect: Rect): List Returns the annotation objects located in the specified map rectangle.
-
func addAnnotations(annotations: List) Adds an array of annotation objects to the map view.
-
func showAnnotations(annotations: List, animated: Bool = true) Sets the visible region so that the map displays the specified annotations.
-
func removeAnnotations(annotations: List) Removes an array of annotation objects from the map view.
-
func selectAnnotation(annotationPoint: MapPointAnnotation, animated: Bool = true) Selects the specified annotation and displays a callout view for it.
-
func deselectAnnotation(annotationPoint: MapPointAnnotation, animated: Bool = true) Deselects the specified annotation and hides its callout view.
-
func animate(duration: Float, delay: Float, options: AnimationOption, animations: Closure, completion: Closure) Animate changes to one or more views using the specified duration, delay, options and completion handler.
-
func setFocus() Force focus to be set to the selected control. For TextField and TextView that means force Keyboard to appear.
-
func clearFocus() Clear focus from selected control
Enums
MapType
- .Hybrid
- .HybridFlyover
- .Satellite
- .SatelliteFlyover
- .Standard
MapUserTrackingMode
- .Follow
- .FollowWithHeading
- .None
AnimationOption
- .AllowAnimatedContent
- .AllowUserInteraction
- .Autoreverse
- .BeginFromCurrentState
- .CurveEaseIn
- .CurveEaseInOut
- .CurveEaseOut
- .CurveLinear
- .LayoutSubviews
- .OverrideInheritedCurve
- .OverrideInheritedDuration
- .OverrideInheritedOptions
- .Repeat
- .ShowHideTransitionViews
- .TransitionCrossDissolve
- .TransitionCurlDown
- .TransitionCurlUp
- .TransitionFlipFromBottom
- .TransitionFlipFromLeft
- .TransitionFlipFromRight
- .TransitionFlipFromTop
- .TransitionNone